How to sell products on the website via PayPal
Table of Contents
Okay, there are two payment options:
- Somebody buys something on your website, you receive the notification by email and after that you can ship the product to the customer (or send it by email).
- Somebody buys something on your website, then the website receives payment notification and automatically send the product to the customer.
I think the second way is absolutely better because it works without you — you can snowboarding or be asleep at this time 🙂
>> Three different WooCommerce hooks after payment completed
>> Create a Payment Gateway Plugin for WooCommerce
So, how to make it work?
Step 1. Everything begins with a form
That’s your payment form, more information about PayPal form parameters you can find here and here.
<form action="https://www.paypal.com/cgi-bin/webscr" method="post"> <input type="hidden" name="cmd" value="_xclick"> <!-- The PayPal account to pay --> <input type="hidden" name="business" id="business" value="YOUR PAYPAL EMAIL HERE" /> <!-- Amount price --> <input type="hidden" name="amount" id="amount" value="9.00" /> <!-- It will be shown on PayPal checkout page --> <input type="hidden" name="item_name" id="item_name" value="ITEM NAME" /> <!-- Specify your product ID here to process it later --> <input type="hidden" name="item_number" id="item_number" value="1038" /> <!-- Charset --> <input type="hidden" name="charset" value="utf-8"> <!-- Thank You page, the customer will be redirected after the payment --> <input type="hidden" name="return" value="https://rudrastyh.com/thank-you"> <button>Buy Now</button> </form>
Paypal IPN (Instant payment notifications) mechanism
Step 2. The IPN settings
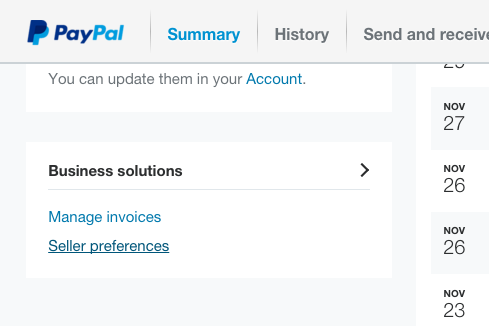
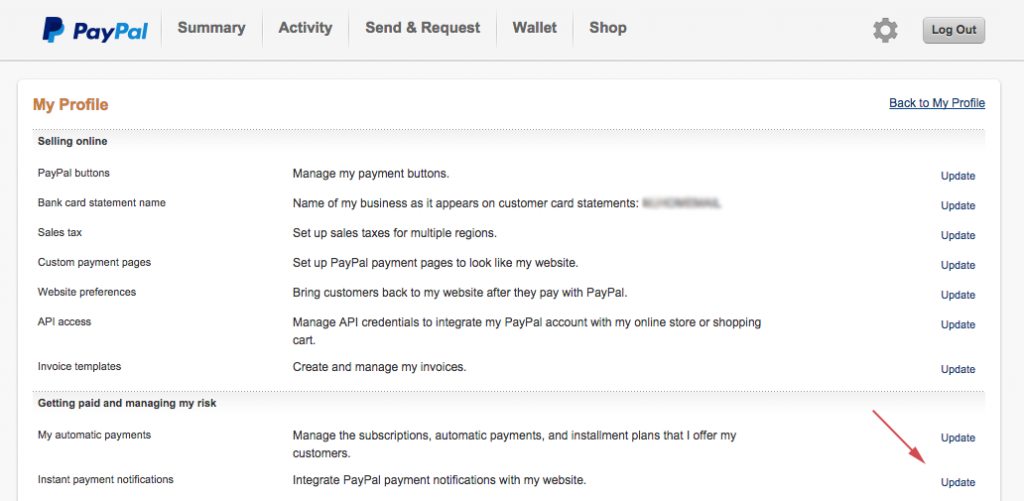
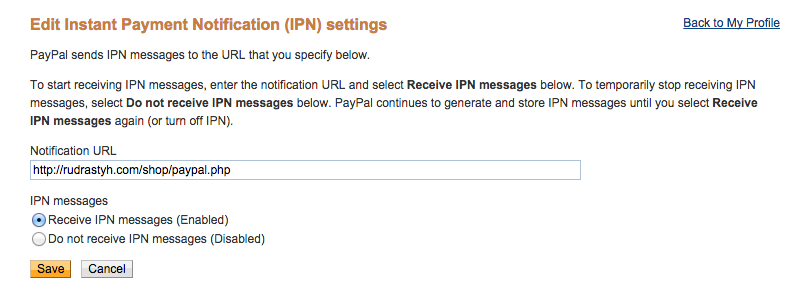
Step 3. The code
The last step is to process PayPal notifications. This is the content of /shop/paypal.php
file.
<?php $r = array( 'status' => $_POST['payment_status'], 'payer_email' => $_POST['payer_email'] ); if( isset( $_POST['item_number'] ) ) { $r['item_number'] = intval($_POST['item_number']); } elseif ( isset( $_POST['item_number1'] ) ) { $r['item_number'] = intval($_POST['item_number1']); } if( strtolower( $_POST['payment_status'] ) == 'completed' && $r['item_number'] ) { /* * On success - do some stuff here, you can: * register new user * send the product by email * redirect customer to a product page * etc */ exit(); } // uncomment the following lines if you want to receive the debug message each time // $headers = "Content-type: text/html; charset=utf-8 \r\n"; // $headers .= "From: paypal.php <[email protected]>\r\n"; // $msg = print_r($_POST, true); // mail("[email protected]", 'IPN debug message', $msg, $headers); exit();