How to remove WordPress default meta boxes
Table of Contents
In the first part of this tutorial I will show you how to remove WordPress default meta boxes
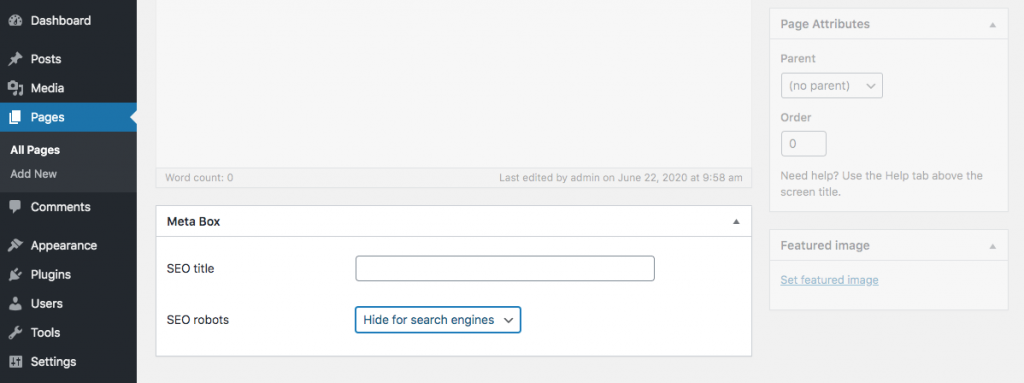
Remove Default Meta Boxes
On every edit post type page WordPress already has predefined meta boxes, you can even see a couple of them on the screenshot above, I mean “Page attributes” and “Featured image” of course.
There are two ways to remove a default metabox – using remove_meta_box()
function or with the help of remove_post_type_support()
.
remove_meta_box()
Straight to the example. Let’s suppose that we have to remove both metaboxes I’ve already mentioned – “Page attributes” and “Featured image”. The code will be:
add_action( 'admin_menu', 'misha_remove_meta_box' ); function misha_remove_meta_box(){ // Featured image remove_meta_box( 'postimagediv', 'page', 'normal' ); // Page attributes remove_meta_box( 'pageparentdiv', 'page', 'normal' ); }
The first parameter of the function is a meta box ID, the easiest way to find out it is to inspect the code in your browser, but just in a case here is the list of default meta boxes IDs.
commentstatusdiv
– Discussion,slugdiv
– Slug,commentsdiv
– Comments,postexcerpt
– Excerpt,authordiv
– Author,revisionsdiv
– Revisions,postcustom
– Custom Fields,trackbacksdiv
– Send Trackbacks,categorydiv
– Categories,tagsdiv-post_tag
– Tags,{Taxonomy name}div
– Any taxonomy,submitdiv
– Publish.
The second parameter of remove_meta_box()
function is a post type you would like to remove it from.
remove_post_type_support()
Let’s try to do the same we did with remove_meta_box() function right now.
add_action( 'init', 'misha_remove_meta_box_2' ); function misha_remove_meta_box_2() { // Featured image remove_post_type_support( 'page', 'thumbnail' ); // Page attributes remove_post_type_support( 'page', 'page-attributes' ); }
As you can see the code is similar, but at the same time is different. I think the most correct way to remove default WordPress meta boxes is with remove_post_type_support()
function, because it actually turns off a specific feature for a specific post type, and remove_meta_box()
function just removes a meta box.
Features / Meta Boxes:
thumbnail
— Featured Image metaboxauthor
— Author metaboxexcerpt
— Excerpt metaboxtrackbacks
— Send trackbacks metaboxcustom-fields
— Custom Fields metaboxcomments
— Comments metaboxrevisions
— Revisions metaboxpage-attributes
— Page Attributes metabox
You can also remove such areas as title or content editor:
title
editor