WordPress allows to use full size image and Image Sizes Explained
Table of Contents
When you upload a picture to your WordPress website, its copies – images with additional sizes will be created. Why do we need them? Because we don’t use the same size image everywhere on the site:
- in posts,
- in portfolio,
- in popups,
- as post thumbnails on archive pages,
- in widgets,
- in administration area etc;
So, for example WordPress allows you to use full size image on posts pages and 150×150 (for example) images on archive pages.
But wait – keep in mind that the more registered image sizes you have, the more files will be on your website and more time will be needed to process images during upload.
Default WordPress Image Sizes
WordPress supports several image sizes by default. You can find and change them in administration area in “Settings > Media”.
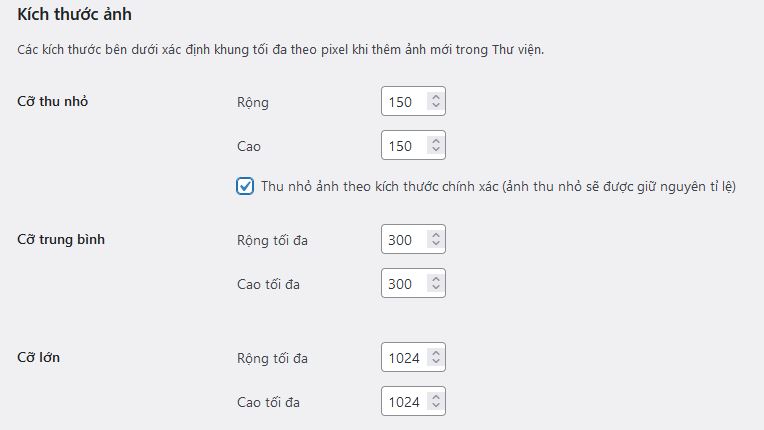
So, for example you can change WordPress thumbnail size on this page.
What if you don’t use them and do not want WordPress to generate a copy for each of these sizes. You can insert the following hook into your theme functions.php
.
But always keep in mind that if your WordPress theme receives updates, then better use a child theme or a custom plugin.
add_filter('intermediate_image_sizes', 'misha_turn_off_default_sizes'); function misha_turn_off_default_sizes( $default_image_sizes) { unset( $default_image_sizes['thumbnail']); // turn off thumbnail size unset( $default_image_sizes['medium']); // turn off medium size unset( $default_image_sizes['large']); // turn off large size return $default_image_sizes; }
medium_large
The medium_large
image size appeared in WordPress 4.4 together with responsive images feature. It has fixed width 768
and proportional height.
You can deactivate medium_large
size as simple as with this code:
add_filter('intermediate_image_sizes', 'misha_deactivate_medium_large'); function misha_deactivate_medium_large( $default_image_sizes ){ $default_image_sizes['medium_large']; return $default_image_sizes; }
How to Get All Image Sizes?
Sometimes you have to find out what is the list of available image sizes on the website. Unfortunately there is no common way to do it for both default and custom images sizes, so:
- If you need the list of default image sizes, you can use
get_intermediate_image_sizes()
, which returns an array with default image sizes, example:
print_r( get_intermediate_image_sizes() ); /* Array ( [0] => thumbnail [1] => medium [2] => medium_large [3] => large ) */
If you have to list registered image sizes, you get them from $_wp_additional_image_sizes
global variable, and I can teel you more, you can also get image dimensions from there, example:
global $_wp_additional_image_sizes; print_r( $_wp_additional_image_sizes ); /* Array ( [my-image-size-name] => Array ( [width] => 400 [height] => 400 [crop] => 1 ) [twentyseventeen-featured-image] => Array ( [width] => 2000 [height] => 1200 [crop] => 1 ) ) */
Functions
It is easy enough to use WP image sizes in administration area – all you have to do is to select an image size you need from the dropdown. Let’s better make a look at functions then.
the_post_thumbnail()
Top 1 in my list, because I use it more often than anything else.
the_post_thumbnail( $size = 'thumbnail', $attr = '' );
Please note, that this function prints an <img>
tag, not a URL.$sizeHere is what you can use as a parameter value:
- image size identifier (name) –
medium
,custom
etc, by defaultthumbnail
, - custom size
array( width, height )
. If you use it, the most appropriate image size will be displayed. - you can pass
full
value to this parameter and an original image will be displayed.
$attrYou can pass any HTML attributes here as an array, you can even rewrite src
attribute!
get_the_post_thumbnail()
This function is very similar to the_post_thumbnail(), the difference is that:
- The first
get_the_post_thumbnail()
is a post ID, everything else is the same, - It returns an image, not prints it.
Example:
echo get_the_post_thumbnail( get_the_ID(), 'medium' );
wp_get_attachment_image_src()
The function allows to get an image URL.
wp_get_attachment_image_src( $attachment_id, $size = 'thumbnail' );
Please note, that an attachment ID is not a post ID. But you can easily get it, for example if you need a featured image ID, you can use get_post_thumbnail_id()
function.
Example:
$image_array = wp_get_attachment_image_src( get_post_thumbnail_id(), 'large' ); echo $image_array[0];
About the $size
parameter and a size array read a little above.